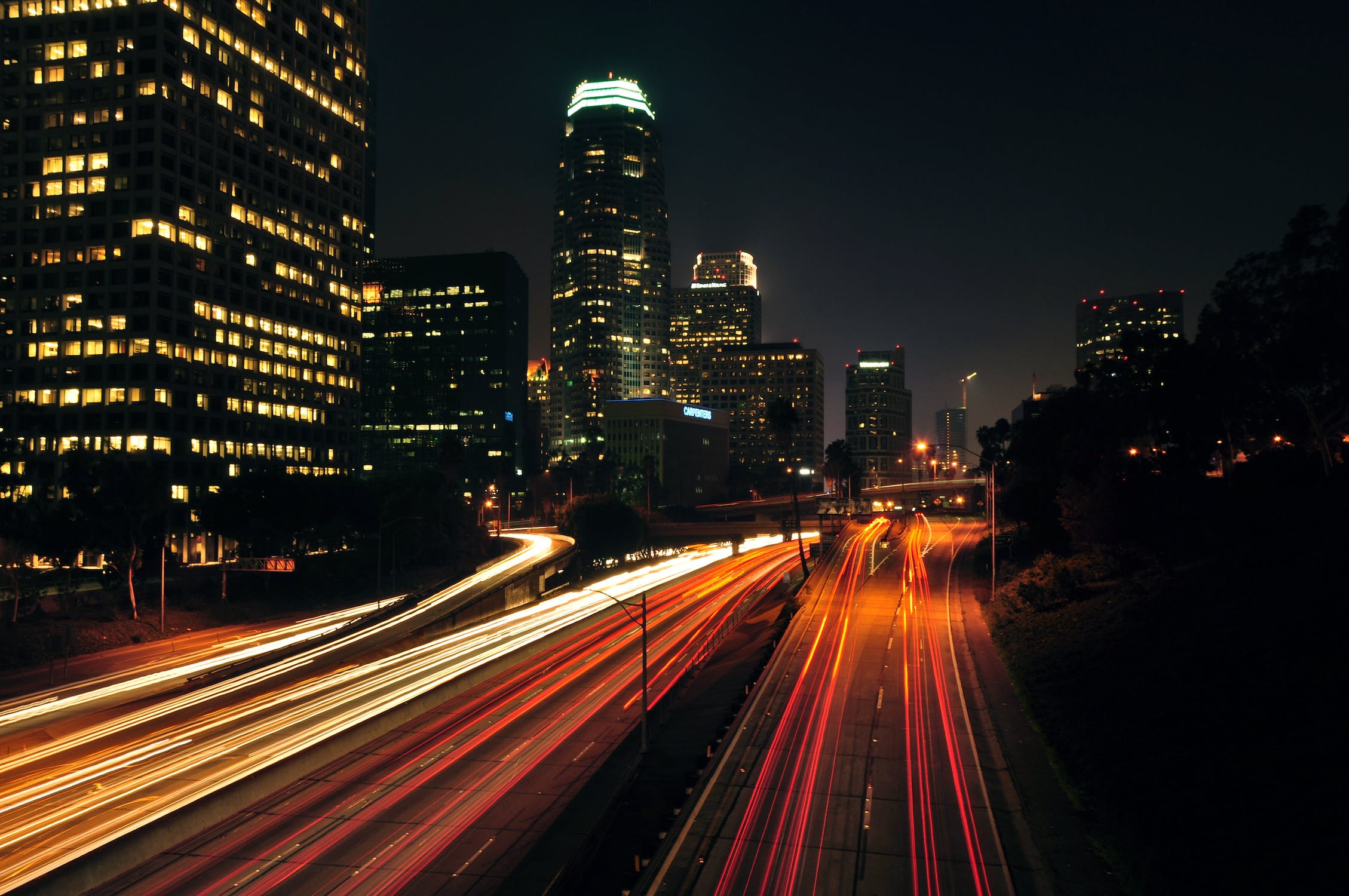
If you’ve ever wondered how Ruby on Rails or any other web framework can talk to a database, this article is for you!
Object Relationship Mapping
An ORM is simply an Object Relationship Mapping, and it abstracts the database away from the object that is talking with it.
With Rails, you can talk with several database types, MYSQL, Postgres, Oracle, SQLLite, etc.
These databases use different SQL standards, regardless of the underlying database, there’s alot needed to build and sanitize queries, parse the returned data set and make it accessible to the developer.
With an ORM, this is all done for you.
Enter Active Record
In Ruby on Rails, Active Record is the library that handles the relationship mapping, and makes it quite easy to define relationships, fields and extra functionality that you wouldn’t get from a pure SQL approach (there’s exceptions to this rule).
Here’s an example of how might query a list of products:
SELECT * FROM products
WHERE name ILIKE 'peanut%';
This on its own will return a string file back of records, but this still needs to be parsed and separated.
With Rails, if you had a product model defined, it’s much easier :
products = Product.where("name ILIKE ?", "peanut%")
products.each do |product|
puts product.name
end
On top of this, you’ll also get all the fields from the table defined as smart method names, making it much easier to inspect and use them in your application development.
Why you shouldn’t use an ORM with Ruby on Rails?
There’s a few scenarios where using the Active Record ORM doesn’t make sense.
SQL queries can get quite complex, with many joins, sub queries, recursive queries, data mapping and analyzing index performance.
In these scenarios, it’s better to use the raw SQL query and map the data yourself.
Fortunately, with Ruby on Rails, the Active Record code provides a lower level abstraction that makes it easy to write custom SQL queries and parse the data.
query = <<-SQL
SELECT id, name, product_code, purchase_count
FROM products
WHERE purchase_count > 100
UNION
SELECT id, name, product_code, purchase_count
FROM archived_products
SQL
records = ActiveRecord::Base.connection.execute(query)
records.each do |record|
puts record["id"]
puts record["name"]
puts record["product_code"]
puts record["purchase_count"]
end
In this example, we combined results from both the products
table and the archived_products
table. Instead of combining them in Ruby, it was easier to use the UNION
operation in SQL and run this as a raw SQL and map the results ourselves.
There are for more complex queries possible, so it’s always good to be able to go back and forth between using the ORM and a query object
You should use an ORM too
While an ORM might be a bit cumbersome to a new developer used to writing SQL queries, it’s an effective way of managing many object types, relationships and interacting with a database. Most languages provide an ORM making easier to perform queries, update data and provide a scalable web application.
With Ruby on Rails, Active Record does a great job of handling talking with numerous database types, abstracting the various database SQL standards and making it super easy to use the data for your web application.